Last week I published an article on “clipping convex hulls”. The article included several randomly generated examples. Every time you refresh the article, you’re given a new set examples based on a new set of randomly generated points.
I thought we could dive into how I used React to generate each of those examples, and how I embedded those React components into a Jekyll-generated static page.
Creating Our Examples
To show off the process, let’s create three React components and embed them into this very article (things are about to get meta). We’ll start by creating a new React project using create-react-app
:
create-react-app examples
cd examples
yarn start
This examples
project will hold all three examples that we’ll eventually embed into our Jekyll-generated article.
The first thing we’ll want to do in our examples
project is to edit public/index.html
and replace the root
div with three new divs, one
, two
, and three
: one to hold each of our examples:
<body>
<noscript>You need to enable JavaScript to run this app.</noscript>
<div id="one"></div>
<div id="two"></div>
<div id="three"></div>
</body>
Next, we’ll need to instruct React to render something into each of these divs. We can do that by editing our src/index.js
file and replacing its contents with this:
import React from 'react';
import ReactDOM from 'react-dom';
import "./index.css";
ReactDOM.render(<One />, document.getElementById('one'));
ReactDOM.render(<Two />, document.getElementById('two'));
ReactDOM.render(<Three />, document.getElementById('three'));
We’ll need to define our One
, Two
, and Three
components. Let’s do that just below our imports. We’ll simply render different colored divs for each of our three examples:
const One = () => (
<div style={{ height: "100%", backgroundColor: "#D7B49E" }} />
);
const Two = () => (
<div style={{ height: "100%", backgroundColor: "#DC602E" }} />
);
const Three = () => (
<div style={{ height: "100%", backgroundColor: "#BC412B" }} />
);
We’re currently telling each of our example components to fill one hundred percent of their parents’ height. Unfortunately, without any additional information, these heights will default to zero. Let’s update our index.css
file to set some working heights for each of our example divs:
html, body {
height: 100%;
margin: 0;
}
#one, #two, #three {
height: 33.33%;
}
If we run our examples
React application, we’ll see each of our colored example divs fill approximately one third of the vertical height of the browser.
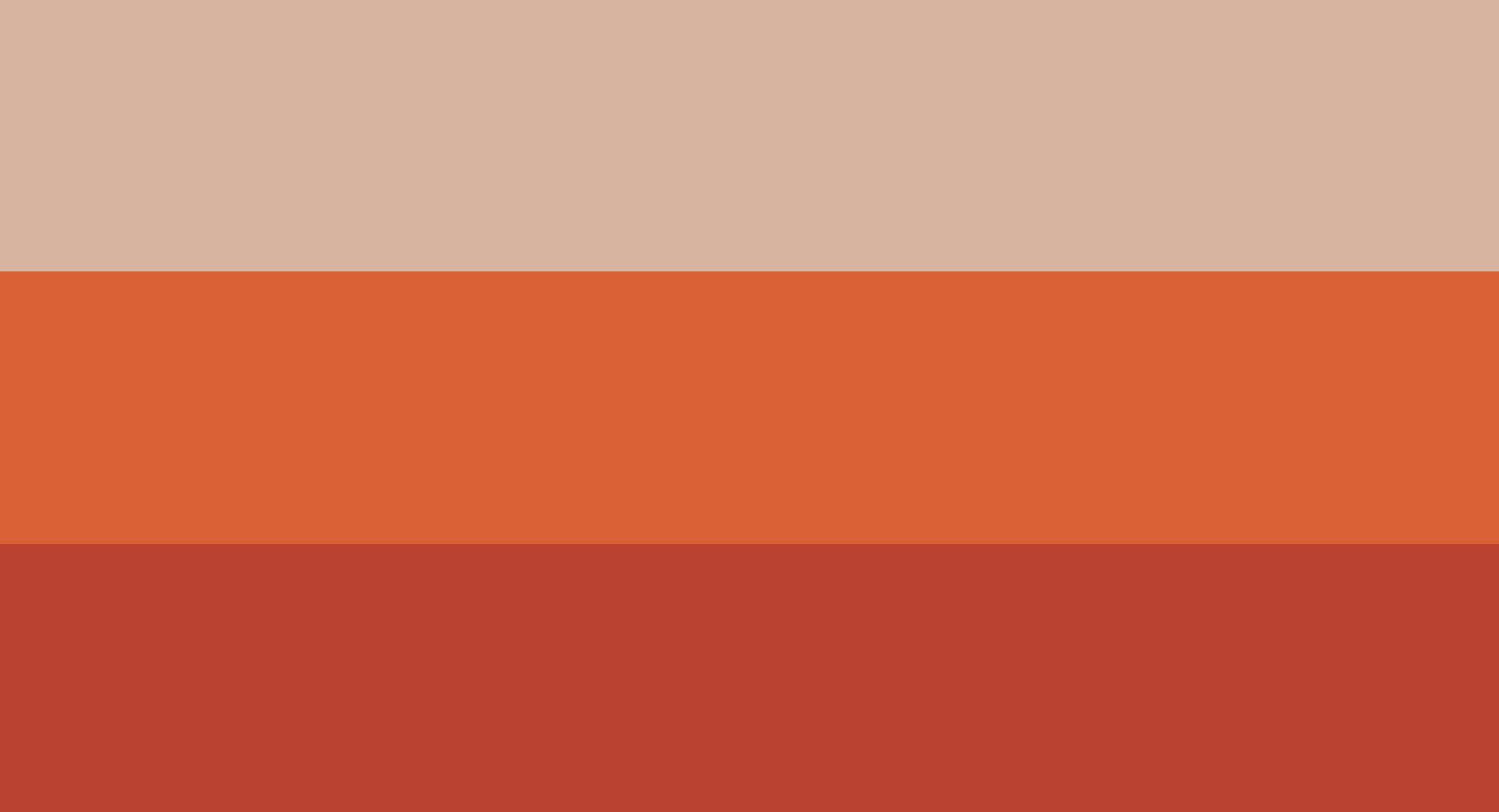
Embedding Our Examples
So now that we’ve generated our example React components, how do we embed them into our Jekyll post?
Before we start embedding our React examples into a Jekyll post, we need a Jekyll post to embed them into. Let’s start by creating a new post in the _posts
folder of our Jekyll blog, called 2019-08-05-embedding-react-components-in-jekyll-posts.markdown
:
---
layout: post
title: "Embedding React Components in Jekyll Posts"
---
Last week I published an article on "clipping convex hulls"...
Great. Now that we have a post, we need to decide where our examples will go. Within our post, we need to insert three divs with identifiers of one
, two
, three
, to match the divs in our examples
React project.
Let’s place one here:
Another here:
And the third just below the following code block that shows off how these divs would appear in our post:
...
Let's place one here:
<div id="one" style="height: 4em;"></div>
Another here:
<div id="two" style="height: 4em;"></div>
...
<div id="three" style="float: right; height: 4em; margin: 0 0 0 1em; width: 4em;"></div>
...
Notice that we’re explicitly setting the heights of our example components, just like we did in our React project. This time, however, we’re setting their heights to 4em
, rather than one third of their parents’ height. Also notice that the third example is floated right with a width of 4em
. Because our React components conform to their parents properties, we’re free to size and position them however we want within our Jekyll post.
At this point, the divs in our post are empty placeholders. We need to embed our React components into the post in order for them to be filled.
Let’s go into our examples
React project and build the project:
yarn build
This creates a build
folder within our examples
project. The build
folder contains the compiled, minified, standalone version of our React application that we’re free to deploy as a static application anywhere on the web.
You’ll notice that the build
folder contains everything needed to deploy our application, including an index.html
file, a favicon.ico
, our bundled CSS, and more. Our Jekyll project already provides all of this groundwork, so we’ll only be needing a small portion of our new build bundle.
Specifically, we want the Javascript files dumped into build/static/js
. We’ll copy the contents of build/static/js
from our examples
React project into a folder called js/2019-08-05-embedding-react-components-in-jekyll-posts
in our Jekyll project.
We should now have three Javascript files and three source map files being served statically by our Jekyll project. The final piece of the embedding puzzle is to include the three scripts at the bottom of our Jekyll post:
<script src="/js/2019-08-05-embedding-react-components-in-jekyll-posts/runtime~main.a8a9905a.js"></script>
<script src="/js/2019-08-05-embedding-react-components-in-jekyll-posts/2.b8c4cbcf.chunk.js"></script>
<script src="/js/2019-08-05-embedding-react-components-in-jekyll-posts/main.2d35bbcc.chunk.js"></script>
Including these scripts executes our React application, which looks for each of our example divs, one
, two
, and three
on the current page and renders our example components into them. Now, when we view our post, we’ll see each of our example components rendered in their full, colorful glory!